The EEPROM is an internal memory of the ESP8266 microcontroller which allows to keep in memory data after restarting the card. When working with microcontrollers, it is interesting to keep in memory data such as the identifier and the password of the Wifi.
Material
- Computer
- NodeMCU ESP8266
- USB A Male/Micro B Male Cable
Principle of operation
The ESP8266 microcontroller has a Flash memory area to simulate the EEPROM of the Arduino. This is a special memory location in the microcontroller where data remains in memory even after the board is turned off. One important thing to note is that the EEPROM has a limited size and life span. The memory cells can be read as many times as necessary but the number of write cycles is limited to 100,000. It is advisable to pay close attention to the size of the stored data and how often you want to update it. The overall flash memory size is usually 4MB. The EEPROM of the ESP8266 has a size of 4kB.
If you want to record real-time data from a fleet of sensors to plot curves, it is best to go for an SD card module to store the data.
Code with the EEPROM library
To interface with the EEPROM of the ESP8266, we can use the EEPROM.h library, very similar to the one for Arduino with some differences. Before using the function, we have to initialize the size of the memory with begin() and the update function does not exist but the write function does something similar.
- begin() to initialize the memory size
- put() to write
- get() to read
- commit() to validate changes
Other functions of the library can be used depending on the use you have of the EEPROM.
//Libraries #include <EEPROM.h>//https://github.com/esp8266/Arduino/blob/master/libraries/EEPROM/EEPROM.h //Constants #define EEPROM_SIZE 12 void setup() { //Init Serial USB Serial.begin(115200); Serial.println(F("Initialize System")); //Init EEPROM EEPROM.begin(EEPROM_SIZE); //Write data into eeprom int address = 0; int boardId = 18; EEPROM.put(address, boardId); address += sizeof(boardId); //update address value float param = 26.5; EEPROM.put(address, param); EEPROM.commit(); //Read data from eeprom address = 0; int readId; EEPROM.get(address, readId); Serial.print("Read Id = "); Serial.println(readId); address += sizeof(readId); //update address value float readParam; EEPROM.get(address, readParam); //readParam=EEPROM.readFloat(address); Serial.print("Read param = "); Serial.println(readParam); EEPROM.end(); } void loop() {}
Result
The read values correspond to the stored values. You can remove the writing part and run the code again to check that the values are well kept in memory.
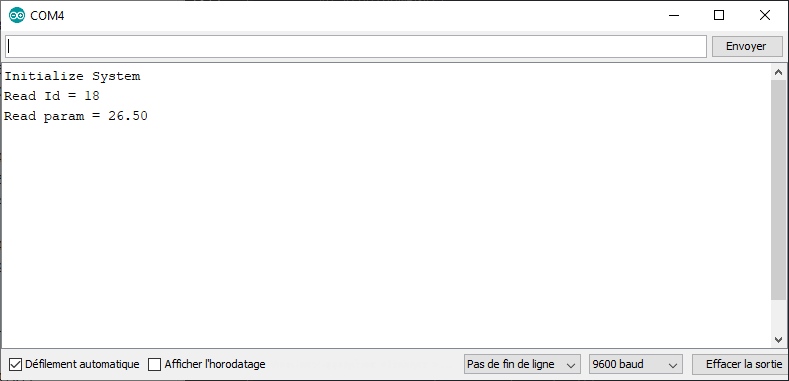
Applications
- Keep in memory the ssid and password of the Wifi network on an ESP8266
Sources
Retrouvez nos tutoriels et d’autres exemples dans notre générateur automatique de code
La Programmerie
I think that should be 4KB and not 4MB??
You’re absolutely right. 4MB is the size of the flash memory. Only 4kB are used for eeprom.
Thanks for letting me know
Thank you so much for the example, it works !!!
Need to try the wifi passowrd storage now 🙂
Great explanation and code! Thanks
Thank you for the example. It tried it on a NodeMCU and it works fine.
But not on an ESP-01, my final target.
On my ESP-01, it appears that the “EEPROM” is written and can be re-read correctly, but after a reset, or a power off / power on sequence, it returns to 00.
I have written: #define EEPROM_SIZE 12, and I try to write and read a single byte.
What is the problem?
Thanks for any help.
Hello,
Did you select the right board?
Did you try changing the eeprom.begin(value)?
What type do you use to store the variable?
I have checked the points you mention. They seem good.
This is what I do in my program (I have to manipulate a single byte):
// libraries:
#include
#define EEPROM_SIZE 12
// declarations:
byte AdresseModule;
// setup:
EEPROM.begin(EEPROM_SIZE);
EEPROM.get(0, AdresseModule);
// writing:
EEPROM.put(0, AdresseModule);
EEPROM.commit();
I have tried read and write instead of get and put. Same result: it works fine on NodeMCU, not on ESP-O1.
Hello thank you for the tutorial. I was ableto get this code working however after a while I kept getting this error.
‘EEPROM_start’ was not declared in this scope
42 | : _sector(((EEPROM_start – 0x40200000) / SPI_FLASH_SEC_SIZE))
It seems to be an error in the library files, but I did not edit anything from those files I downloaded from github.
Did you update the library or IDE version?
https://github.com/esp8266/Arduino/issues/6531
My arduino ide version is 1.8.20 and library version is 3.0.2
‘EEPROM_start’ was not declared in this scope
42 | : _sector(((EEPROM_start – 0x40200000) / SPI_FLASH_SEC_SIZE))
I still get this error
My Arduino IDE Version is 1.8.20, and library I used is the one provided in this link
//https://github.com/esp8266/Arduino/blob/master/libraries/EEPROM/EEPROM.h
My arduino ide is 1.8.20, and my library I used is from this link //https://github.com/esp8266/Arduino/blob/master/libraries/EEPROM/EEPROM.h
It says version 3.0.2 on github.
My arduino ide is 1.8.20, and my library I used is from this link //https://github.com/esp8266/Arduino/blob/master/libraries/EEPROM/EEPROM.h
It says version 3.0.2 on github.
‘EEPROM_start’ was not declared in this scope
42 | : _sector(((EEPROM_start – 0x40200000) / SPI_FLASH_SEC_SIZE))
This error still shows up
Hello,
Thanks for the article.
In Arduino’s we can use eeprom.update() to let only different value from the previous to be saved, so it can extend lifespan of eeprom.
In ESP8266, code is not available, we can only use eeprom.write() and eeprom.put().
Can someone tell me how to interface eeprom.update() in ESP8266 like Arduino does?
Thanks a lot,
According to ESP8266 EEPROM:write() function, it does check if the value is different
hello can you help me with this sketch
https://pastebin.com/rr7reJWP
the idea is i want to save the slider value and the button value so when there is blackout or the esp restart the code will load the input value. and run automatically.
i success upload the sketch but when boot an error occurs
————— CUT HERE FOR EXCEPTION DECODER —————
Exception (3):
epc1=0x40201388 epc2=0x00000000 epc3=0x00000000 excvaddr=0x40201028 depc=0x00000000
>>>stack>>>
ctx: cont
sp: 3ffffdf0 end: 3fffffc0 offset: 0190
3fffff80: 3fffdad0 3ffeec54 3ffeec1c 40201365
3fffff90: feefeffe feefeffe feefeffe feefeffe
3fffffa0: 3fffdad0 00000000 3ffeed84 402078f0
3fffffb0: feefeffe feefeffe 3ffe85e8 40100d35
<<<stack<<<
————— CUT HERE FOR EXCEPTION DECODER —————
ets Jan 8 2013,rst cause:2, boot mode:(3,6)
load 0x4010f000, len 3460, room 16
tail 4
chksum 0xcc
load 0x3fff20b8, len 40, room 4
tail 4
chksum 0xc9
csum 0xc9
v0004a210
~ld
please help me
Simple tutorial, however everything works with address 0. please try with different address, it is not working. same code.
very good article. thank alot.